Write a Custom Ruby Function for CSML
A few days ago we went through the process of writing a custom function in Javascript for CSML.
Today we're going to code a Ruby function for CSML. This function will allow you to use your favorite Gem dependencies and Ruby programs in your CSML chatbot!
The use case
We would like to create a chatbot that can fetch the value of your favorite crypto-currencies and convert it into another one.
For instance, we would want to know how much a Bitcoin is worth in Dollars or Ethereum in Litecoins.
To get all the values, we'll use this API.
The Ruby code
IMPORTANT: First, make sure that your version of Ruby is either 2.5 or 2.7, which are the supported versions. If needed, you can have a look at rbenv, an env manager for Ruby (it is a good practice to use it regardless of the use case.)
A. The function
As mentioned in a previous article, custom CSML functions are very similar to Lambda functions:
- They get their parameters from the
event
object that is injected automatically. - They can return values such as
Object
,Array
,String
,Integer
,Float
,Boolean
,null
- The handler (the function that will be triggered) name must be specified (
handler
as default)
You can find CSML custom function boilerplates on Github.
This is for example what a function that returns Hello, {{name}}
looks like:
def handler(event:, context:)
"Hello #{event["name"]}"
end
B. Fetching data from the API
Now that we know how to make a ruby function, let's fetch the datas from the API.
First, we need to set up httparty as a dependency in the current directory. To do so, follow these steps
1- Create the Gemfile
source "https://rubygems.org"
gem "httparty", "~> 0.17.3"
2- Set the Bundle directory and Install the dependency
$ bundle config set path 'vendor/bundle'
$ bundle install
And now we just need to fetch the datas from the API using Ruby with httparty:
require 'httparty'
require 'json'
def handler(event:, context:)
obj = HTTParty.get("https://api.cryptonator.com/api/ticker/#{event["from"]}-#{event["to"]}")
JSON.parse(obj.body)
end
C. Let's package it
Let's now package the function to upload it in CSML Studio
$ zip -r9 myArchive.zip index.rb vendor
Done!
Custom Ruby functions in CSML Studio
Now that we have the packaged function, let's set it up in CSML Studio.
Go to Functions
> Add Custom Functions
> Quick mode
First, we upload the packaged function myArchive.zip
, and fill the form:
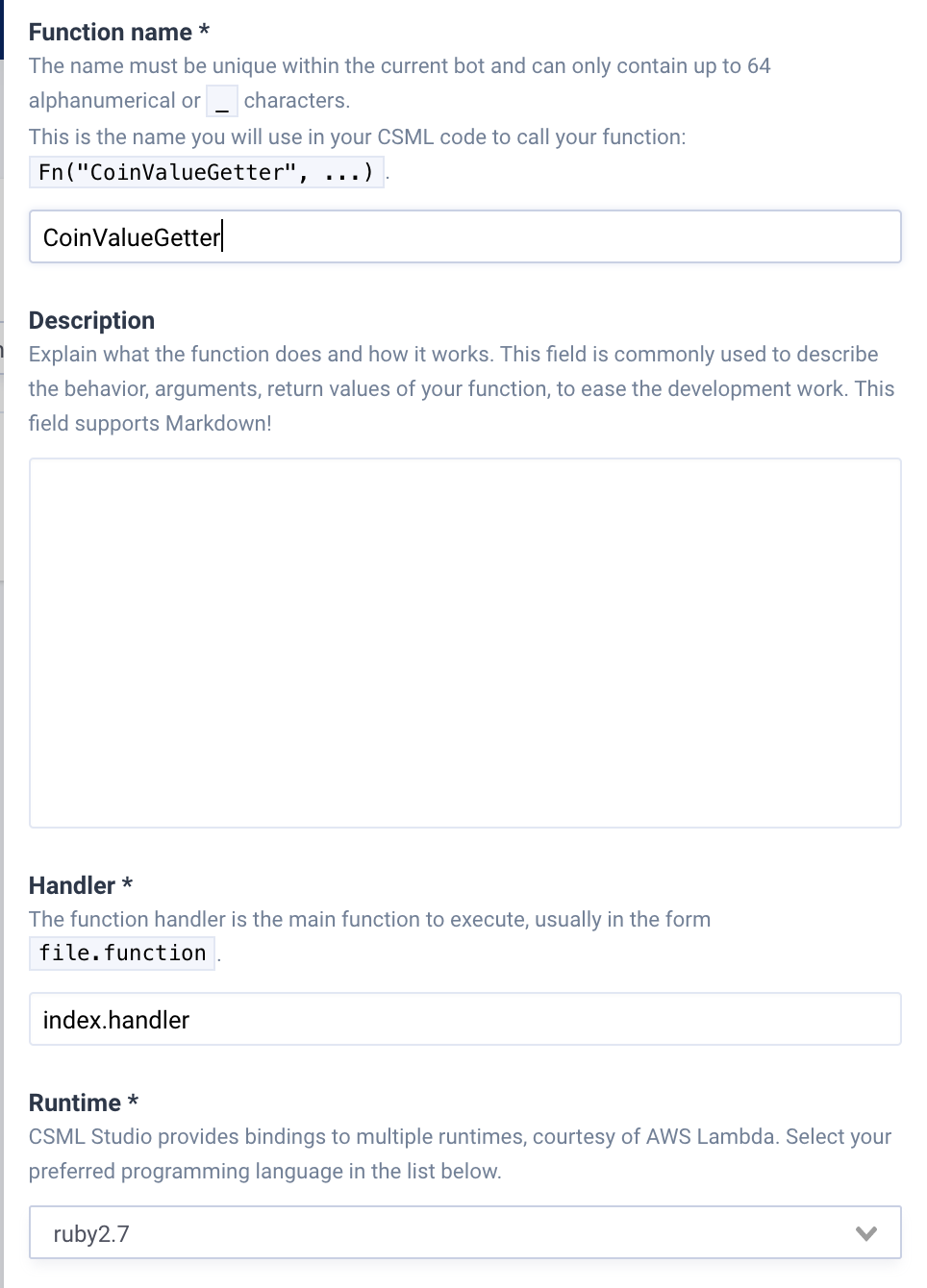
- We give it a name:
CoinValueGetter
- We set the handler as
index.handler
: the function calledhandler
is in theindex.rb
file. - We can set the parameters as
from
andto
, this is not mandatory - We don't need any environment variables, so let's leave this field blank
Click on OK. You are all set!
Now let's use this function in a flow.
start:
say "What coin do you want to check? (btc, eth, ...)"
hold
remember from = event
say "How do you want me to give you the value ? (eur, usd, ltc, ...)"
hold
remember to = event
do val = Fn("CoinValueGetter", from=from, to=to)
say "1{{from}} = {{val.ticker.price}}{{to}}"
goto end
That's it, we've done it! Now we can use a function anywhere in our chatbot!
You can find the entire source code of the function over here: https://github.com/bastienbot/ruby-crypto-price-function