Supercharge your Chatbot with Huggingface Models
If you've built a chatbot before, you must know be now that creating the chatbot is not only a matter of building a nice decision tree, there is much more to it: UX, database, custom API connections, intent classification, deployment, etc...
In most cases CSML has what you need ready out of the box: either within the language or available in CSML Studio. But there a are a few features that require real expertise in a specific field. Huggingface provides a large range of machine learning models available through their very famous and performing product: Transformers. With Huggingface models, you can supercharge your CSML chatbot and get really outstanding user experiences, thanks to all their models available off the shelf: Question Answering, Sentiment Analysis, Summarization, etc...
There are a few ways to use Transformers, you can use it in your local environment, with AWS Sagemaker, or with Huggingface Accelerated Inference API.
In this exemple, we'll use the API and a sentiment analysis model to analyse a user input and adapt the chatbot response depending on how good the user feels.
Get started with Huggingface
First, we'll need to create an account on huggingface.co, they offer a free plan that is more than enough for most use cases.
Once you've created your account, let's head over to the model section to find the perfect model for our conversation! As a reminder, we want to find a Sentiment Analysis model in english. This one seems to to just the job: nlptown/bert-base-multilingual-uncased-sentiment! Bert is a state of the art model that was initially crafted by Google.
Let's find our API key, you click on the menu, and API Key, or just head over to this page: https://api-inference.huggingface.co/dashboard/api_token.
We've got our model, we've got our API key, let's start coding!
Coding the conversation
Coding this conversation is pretty easy, we ask the user how he feels, and we provide one of three different answers based on how the user feels.
start:
say "How are you feeling ?"
hold
// feeling okay
say "I guess this is just a regular day for you..."
// feeling good
say "I'm glad you'd feeling good, I'm feeling great myself! 😎"
//feeling bad
say "Well I guess we all have our ups and downs"
goto end
From here, we need to create a function that is going to query the Huggingface API and return a nice sentiment analysis response so we could call it like this: do sentiment = sentiment_analysis(event)
just after the hold
.
This is the python code that Huggingface provides to perform this HTTP request:
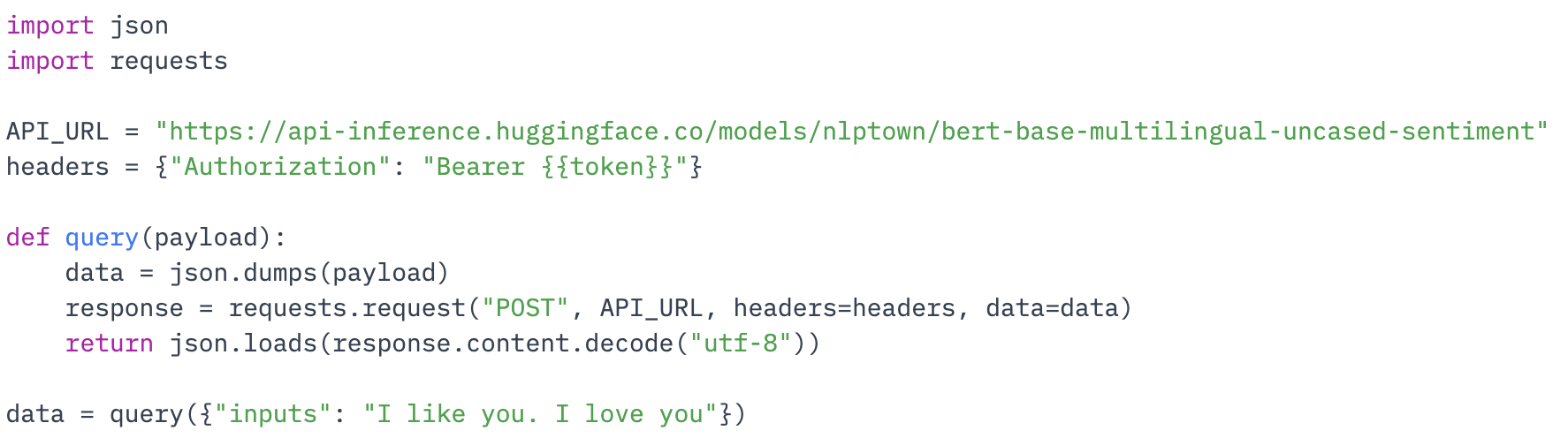
In CSML, it translates to this:
do res = HTTP("https://api-inference.huggingface.co/models/nlptown/bert-base-multilingual-uncased-sentiment")
.set({"Authorization": "Bearer {{token}}"})
.post({"inputs": text})
.send()
I know...CSML just so happen to be easier to read than python, it's always nice to keep that in mind 😎
This HTTP request returns this sort of response:
[
[
{"label":"1 star","score":0.002195029752328992},
{"label":"2 stars","score":0.0022533712908625603},
{"label":"3 stars","score":0.015475977212190628},
{"label":"4 stars","score":0.19356276094913483},
{"label":"5 stars","score":0.7865128517150879}
]
]
Let's create a function, put the HTTP request right at the beginning and process the response so our function will return either:
1
of the user feels bad3
if the user is okay5
if the user is feeling great. You can obviously keep the 5 rating if you like :)
Here is the final function:
fn sentiment_analysis(text):
do res = HTTP("https://api-inference.huggingface.co/models/nlptown/bert-base-multilingual-uncased-sentiment")
.set({"Authorization": "Bearer {{token}}"})
.post({"inputs": text})
.send()
do sentiment = null
if (res) {
foreach (s) in res[0] {
if (!sentiment || s.score > sentiment.score)
do sentiment = s
}
}
if (!sentiment || sentiment.label == "3 stars") return 3
if (sentiment.label.match_regex("[45] stars")) return 5
if (sentiment.label.match_regex("[12] stars")) return 1
return sentiment
And finally we just adapt the flow so we call the function and check what it returns to pick the right answer.
You can check out the entire flow over in this playground: https://play.csml.dev/bot/8da437c5-35b6-4f22-8801-30f2471a054d
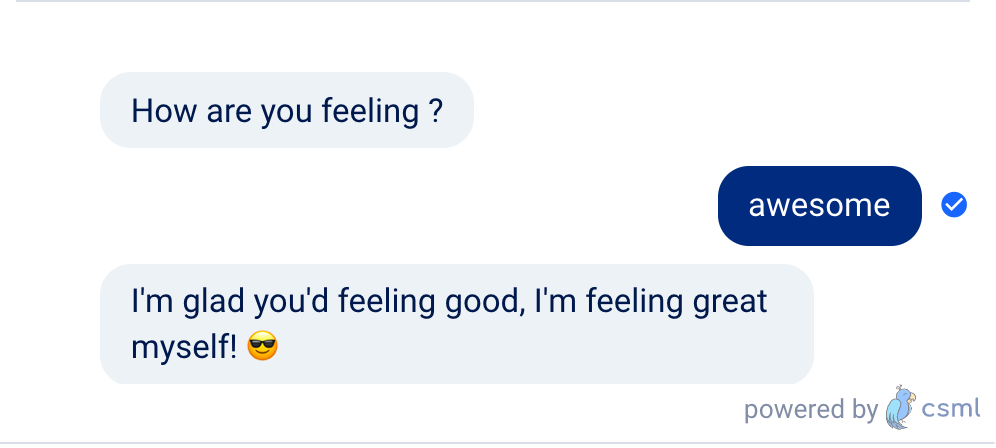
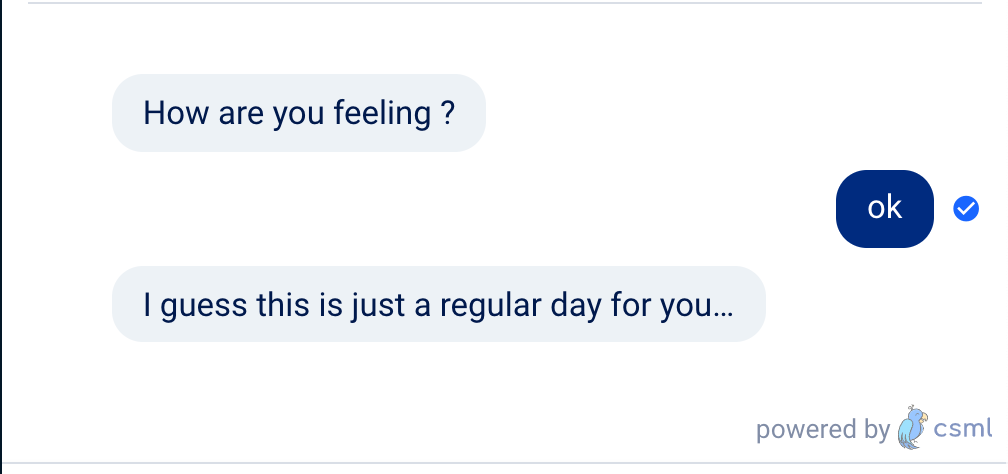
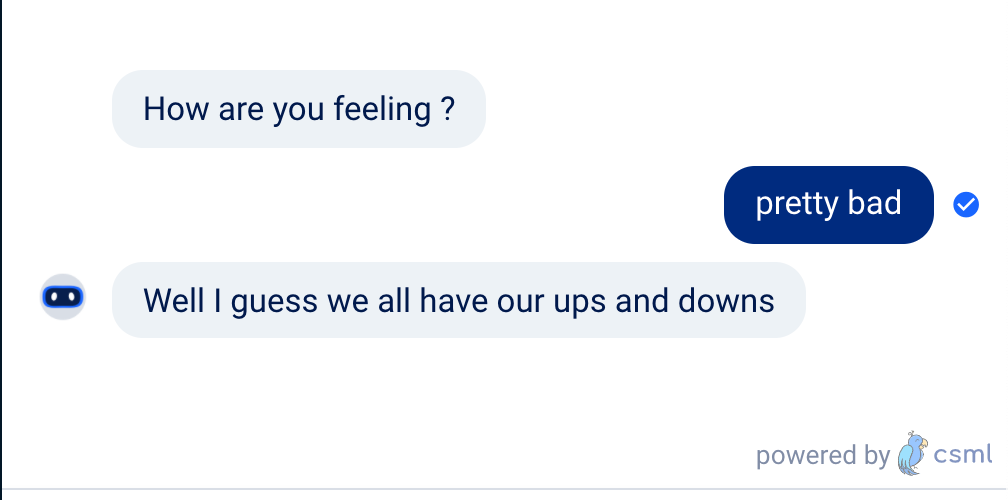
Have fun making great chatbots!